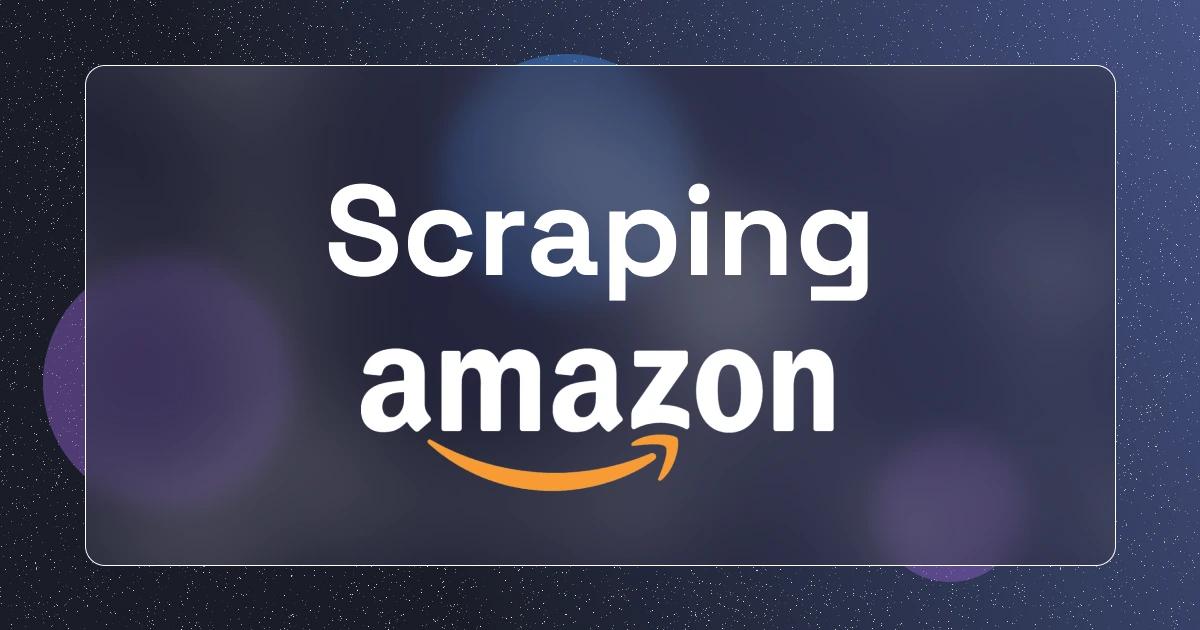
How to Scrape Amazon Product Data in 2025?
Amazon isn’t just the go-to marketplace for online shopping, it’s a treasure trove of insights for businesses. Whether you’re tracking prices, monitoring trends, or researching competitors, Amazon’s data can help you stay ahead. But how do you get this data efficiently without spending hours manually copying and pasting?
In this guide, we’ll take you step by step through the process of extracting data from Amazon. We’ll cover everything from the tools you need to practical examples. If coding isn’t your thing, don’t worry, we’ll also introduce a smarter, automated solution to save you time and effort.
Challenges of scraping Amazon and how to overcome them
Scraping Amazon isn’t as easy as copying text from a web page. The platform has robust systems to prevent bots from accessing its data. Here are some of the main challenges you’ll face:
- Dynamic content: Many product details, like prices and reviews, are loaded using JavaScript. A simple scraper might not even see these elements.
- CAPTCHAs: If Amazon suspects you’re a bot, you’ll face CAPTCHAs that block your access.
- IP blocking: Too many requests from the same IP address can result in your scraper being locked out.
How to overcome these challenges ?
- Use rotating proxies: This makes your requests appear to come from different locations, reducing the chance of detection.
- Employ CAPTCHA-solving tools: These can automate the process of solving CAPTCHAs when they appear.
- Leverage tools like Selenium or Puppeteer: These render JavaScript-heavy pages, ensuring you capture all the necessary details.
Navigating these obstacles takes planning and the right tools, but with a structured approach, it’s entirely possible to collect the data you need.
Tools and techniques for scraping Amazon effectively
To scrape Amazon, you need a combination of tools and techniques to work around its defenses. Here’s a simple roadmap:
1. Set up your environment
Start with Python and the following libraries:
pip install requests beautifulsoup4 lxml
These tools allow you to send requests and parse HTML.
2. Inspect the web page
Use browser developer tools (right-click → Inspect) to find the HTML elements containing product details. For example, product titles are often in a <span>
tag with a class like a-size-medium
.
3. Write a scraper
A simple example to extract titles and prices:
import requests
from bs4 import BeautifulSoup
# Target Amazon product page
url = "https://www.amazon.com/s?k=wireless+headphones"
# Send an HTTP request
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(url, headers=headers)
# Parse the HTML
soup = BeautifulSoup(response.content, "lxml")
# Extract product titles and prices
for product in soup.select(".s-main-slot .s-result-item"):
title = product.select_one("#productTitle")
price = product.select_one(".a-price-whole")
if title and price:
print(f"Product: {title.text.strip()}")
print(f"Price: {price.text.strip()}")
This script fetches search results for "wireless headphones" and prints their titles and prices.
4. Overcome common anti-scraping obstacles
To prevent issues like IP blocking or CAPTCHAs:
- Use a proxy service like Bright Data to rotate IPs.
- Introduce random delays between requests to mimic human behavior.
- For dynamic content, use Selenium to load JavaScript elements.
To prevent getting your IP banned, you can introduce a delay like this:
import time
# Delay between requests
time.sleep(2)
Or even better, make the delay between requests random.
5. Render dynamic content
Some Amazon data, like reviews or images, is loaded dynamically with JavaScript. For these cases, use a tool like Selenium to interact with the page:
from selenium import webdriver
# Set up Selenium WebDriver
driver = webdriver.Chrome()
driver.get("https://www.amazon.com/s?k=wireless+headphones")
# Extract data
titles = driver.find_elements_by_css_selector("#productTitle")
for title in titles:
print(title.text)
driver.quit()
6. Save and analyze your data
Store your results in a structured format for easy analysis. For example, save them as a CSV file:
import csv
with open("products.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerow(["Product", "Price"])
for product in soup.select(".s-main-slot .s-result-item"):
title = product.select_one("#productTitle")
price = product.select_one(".a-price-whole")
if title and price:
writer.writerow([title.text.strip(), price.text.strip()])
These tools and techniques give you a solid foundation to scrape Amazon efficiently while staying mindful of its challenges.
Alternatives to manual scraping for Amazon data
If writing and maintaining a custom scraper for Amazon sounds too complex or time-consuming, there are alternatives that can simplify the process. These options provide structured access to Amazon data without requiring deep technical knowledge or constant updates to handle website changes.
1. Amazon Product Advertising API
Amazon’s official API offers a reliable way to access product data like titles, prices, and reviews.
- Pros: Stable, accurate, and compliant with Amazon’s policies.
- Cons: Limited access for new users, strict usage quotas, and requires approval.
Example: Retrieve product details in Python:
import boto3
# Set up your Amazon Product Advertising API credentials
client = boto3.client(
'product-advertising-api',
aws_access_key_id='YOUR_ACCESS_KEY',
aws_secret_access_key='YOUR_SECRET_KEY',
region_name='us-east-1'
)
# Example request to get product details
response = client.search_items(
Keywords='wireless headphones',
Resources=['ItemInfo.Title', 'Offers.Listings.Price']
)
for item in response['Items']:
print(f"Title: {item['ItemInfo']['Title']['DisplayValue']}")
print(f"Price: {item['Offers']['Listings'][0]['Price']['DisplayAmount']}")
These tools and techniques give you a solid foundation to scrape Amazon efficiently while staying mindful of its challenges.
Alternatives to manual scraping for Amazon data
If writing and maintaining a custom scraper for Amazon sounds too complex or time-consuming, there are alternatives that can simplify the process. These options provide structured access to Amazon data without requiring deep technical knowledge or constant updates to handle website changes.
1. Amazon Product Advertising API
Amazon’s official API offers a reliable way to access product data like titles, prices, and reviews.
- Pros: Stable, accurate, and compliant with Amazon’s policies.
- Cons: Limited access for new users, strict usage quotas, and requires approval.
Example: Retrieve product details in Python:
import boto3
# Set up your Amazon Product Advertising API credentials
client = boto3.client(
'product-advertising-api',
aws_access_key_id='YOUR_ACCESS_KEY',
aws_secret_access_key='YOUR_SECRET_KEY',
region_name='us-east-1'
)
# Example request to get product details
response = client.search_items(
Keywords='wireless headphones',
Resources=['ItemInfo.Title', 'Offers.Listings.Price']
)
for item in response['Items']:
print(f"Title: {item['ItemInfo']['Title']['DisplayValue']}")
print(f"Price: {item['Offers']['Listings'][0]['Price']['DisplayAmount']}")
2. Third-party scraping tools and services
Platforms like ScraperAPI and Bright Data simplify scraping by handling IP rotation, CAPTCHAs, and data collection.
- ScraperAPI: A user-friendly solution that rotates proxies automatically.
- Bright Data: A premium service for high-volume and complex data needs.
Example: Use ScraperAPI to fetch Amazon HTML:
import requests
url = "https://www.amazon.com/s?k=wireless+headphones"
proxy_url = f"https://api.scraperapi.com/?api_key=YOUR_API_KEY&url={url}"
response = requests.get(proxy_url)
print(response.text) # The HTML content of the Amazon page
3. Product Fetcher: A powerful automated solution
For businesses and marketers who want simplicity, Product Fetcher offers a ready-to-use AI-Powered scraping API for retrieving clean, structured Amazon product data.
Why choose Product Fetcher?
- No setup required: Works out of the box without needing to configure scrapers for specific websites.
- Adaptive to site changes: Automatically adjusts to changes in a website’s structure, so you don’t have to worry about maintaining or updating code.
- Handles dynamic content seamlessly: Processes JavaScript-heavy sites without requiring manual intervention.
- No coding necessary: Perfect for non-developers or teams without technical expertise.
- Supports multiple platforms: Easily scales to extract data from Amazon and other major e-commerce websites.
- Reliable: Provides consistently accurate, formatted data like product names, prices, reviews, and images.
Example: Fetch data using Product Fetcher:
curl -X GET "https://product-fetcher.com/api/product?apiKey=${yourApiKey}&url=productUrl"
Or integrate it into your application:
import requests
response = requests.get("https://product-fetcher.com/api/product", params={
"apiKey": "yourApiKey",
"url": "https://www.amazon.com/dp/B09XXXXXXX"
})
data = response.json()
print(data)
This provides data like:
{
"name": "Wireless Headphones",
"price": "49.99",
"currency": "USD",
"rating": "4.5",
"reviews": "152",
"images": ["https://media.com/image.png"],
"sku": "SOMESKU123"
}
How to make the most of your collected Amazon data
Once you’ve collected Amazon product data, the key is transforming it into actionable insights. Here’s how to effectively use your data:
1. Organize your data for easy access
Keeping your data structured is essential for analysis. Store it in:
- Spreadsheets: Tools like Excel or Google Sheets work well for smaller datasets.
- Databases: Relational databases (e.g., PostgreSQL) are ideal for larger datasets, offering better scalability and querying options.
- Dashboards: Visualization tools like Tableau or Power BI can help you monitor data in real time.
2. Analyze pricing strategies
Pricing is one of the most valuable insights Amazon data can provide:
- Compare competitors’ prices: See how your products stand against similar listings.
- Identify discounts and trends: Monitor seasonal sales and discount patterns to inform your pricing strategy.
- Set optimal prices: Use trends to determine pricing that maximizes profit while staying competitive.
3. Evaluate product demand
Use collected data to assess:
- Top-selling categories: Identify the types of products in high demand.
- Trending items: Spot items that are gaining popularity based on reviews or sudden price increases.
- Market gaps: Discover niches where customer demand exceeds available supply.
4. Automate your dropshipping strategy with Amazon data
For dropshippers, automation is key to scaling efficiently. By leveraging Amazon data, you can streamline decision-making and maximize profitability:
- Find products automatically: Use scraped data to identify trending products with high demand and favorable reviews.
- Monitor price fluctuations: Set up automated alerts for price changes, ensuring you always sell at a competitive margin.
- Sync inventory: Automate inventory tracking to match supplier stock levels and avoid overselling.
5. Simplify affiliate marketing websites workflow
Affiliate marketers can save time and improve performance by automating data collection and content creation:
- Dynamic content updates: Use scraped data to automatically refresh product prices and availability in your blog posts or landing pages.
- Personalized recommendations: Analyze customer reviews and ratings to curate the best products for your audience.
- Track performance effortlessly: Automate tracking of affiliate links and commissions to identify top-performing products.
Start growing your business with Amazon data today
Amazon’s data is a powerful resource for businesses, whether you’re refining pricing strategies, scaling your dropshipping store, or enhancing affiliate campaigns. While manual scraping can be effective, it requires constant updates and technical expertise.
Product Fetcher eliminates the hassle, offering an automated way to extract and format data instantly. No setup, no coding, no worries.
Why wait? Try Product Fetcher today FOR FREE and turn Amazon’s data into actionable insights for your business.
Frequently Asked Questions (FAQ)
Share on social